python创建和遍历二叉树,可以使用递归的方式。
例如:
复制代码 代码示例:
#!/usr/bin/python
class node():
def __init__(self,k=None,l=None,r=None):
self.key=k;
self.left=l;
self.right=r;
def create(root):
a=raw_input('enter a key:');
if a is '#':
root=None;
else:
root=node(k=a);
root.left=create(root.left);
root.right=create(root.right);
return root;
def preorder(root): #前序遍历
if root is None:
return ;
else :
print root.key;
preorder(root.left);
preorder(root.right);
def inorder(root): #中序遍历
if root is None:
return ;
else:
inorder(root.left);
print root.key;
inorder(root.right);
def postorder(root): # 后序遍历
if root is None:
return ;
else :
postorder(root.left);
postorder(root.right);
print root.key;
root=None; # 测试代码
root=create(root);
preorder(root);
inorder(root);
postorder(root);
运行程序,建立二叉树如图:
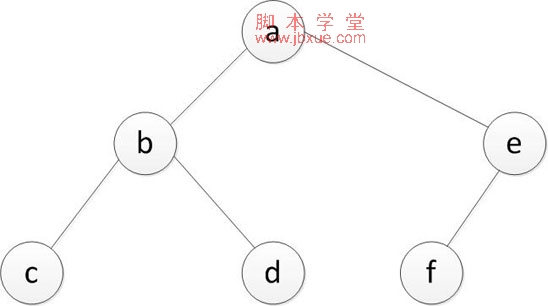
前序遍历结果为: a b c d e f
中序遍历结果为:c b d a f e
后序遍历结果为:c d b f e a